Test driven development for Infrastructure as Code using Pulumi and Jest
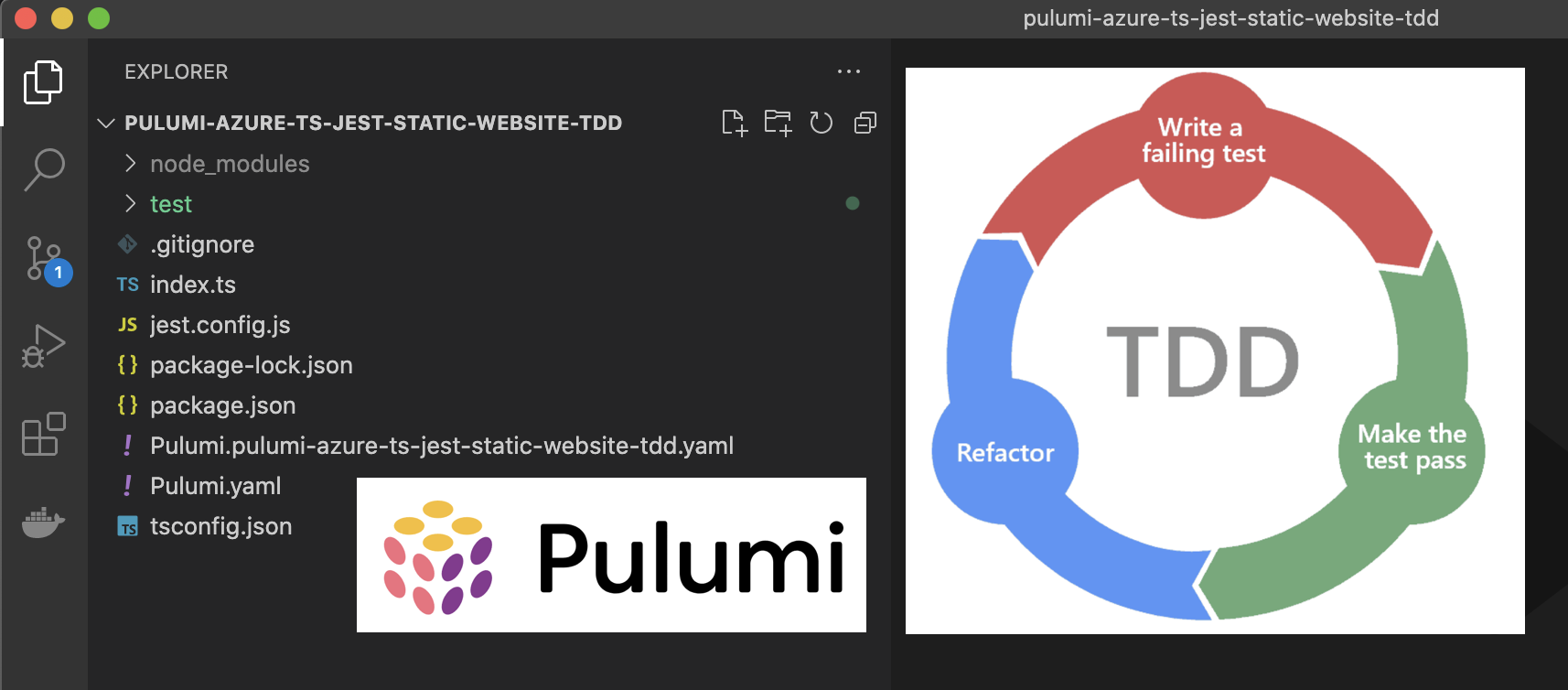
I was always looking for ways to apply TDD while doing Infrastructure as Code development.
Especially when developing a library of reusable components and the code base increases, regressions become inevitable without proper test coverage.
When I started with Terraform a couple of years ago, I was missing the possibility to use familiar programming languages and TDD for IaC development.
Then Pulumi emerged and delivered on the promise to make IaC development possible with widely used programming languages like Typescript, Go, Python, C#.
The Goal
We want to host a static website (also used to host this blog) with a custom domain on Azure in a secure, fast and cost efficient way by using a Storage account with Static Website feature enabled.
flowchart LR User(User) -- https://mydomain.com --> CDN(Azure CDN) subgraph Azure CDN --> BlobContainer subgraph StorageAccount BlobContainer --> StaticWebsite(Static Website Content) end end Pulumi -- Provision --> Azure style StorageAccount fill:#59c style Azure fill:#59c
Pulumi has a great tutorial on how to create exactly this, but we want to do it test driven.
In this post I will be focusing on doing TDD with Pulumi using Typescript and the Jest Framework.
flowchart LR FailingTest(Write a failing test) --> PassingTest(Make the test pass) PassingTest --> Refactor Refactor -->FailingTest style FailingTest fill:#f55 style PassingTest fill:#5d5 style Refactor fill:#59f
If you are using Mocha as test framework, the same priciples apply and you can have a look at the Mocha config in the Pulumi documentation on unit testing
Let’s go!
The complete source code for the article can be found on GitHub: https://github.com/codingarchitect-wq/pulumi-azure-ts-jest-static-website-tdd
First, let’s create a new pulumi project:
pulumi new azure-typescript --dir pulumi-azure-ts-jest-static-website-tdd
cd pulumi-azure-ts-jest-static-website-tdd
The Pulumi new project template will generate some code in the index.ts
file.
Let’s delete that generated code so we have a clean start.
Before:
After:
Open the project in Visual Studio Code
I use Visual Studio Code as an IDE, let’s open our project using it.
code .
Prepare for writing our tests
Before we can start writing our first test, we need to setup Jest
for Typescript.
ts-jest
is a TypeScript preprocessor for jest, that lets you use jest to test projects written in TypeScript.
But first we need to install some additional dependencies for this. We need jest
, ts-jest
and the typings for jest because jest is written in JavaScript. For this we enter the following command:
npm i -D jest ts-jest @types/jest
Next, we need to tell jest that we want to use ts-jest
as a preprocessor. For this we let ts-jest
create a configuration file with the following command:
npx ts-jest config:init
This will create a file named jest.config.js
with a setting for jest
to use the preprocessor js-test
.
Add build and unit-test commands
We still need to add some commands in our package.json, so that npm knows how to build the project and how to run the unit tests.
Add the following command to the package.json:
"scripts": {
"build": "tsc",
"unit-tests": "jest"
}
How Pulumi Mocks work
Pulumi Runtime exposes a way to intercept calls to the provider and mock the results on new resource constructor call (e.g. new azure.storage.Account) and provider function call (e.g. aws.get_availability_zones).
flowchart TB subgraph LanguageHost index.test.js --> index.ts end index.ts -- 1. new Resource --> PulumiEngine subgraph Providers direction TB Azure AWS Kubernetes end PulumiEngine(Pulumi Engine) -- 2. Create/Call --> PulumiMocks(PulumiMocks) PulumiMocks -- X --> Providers PulumiMocks -- 3. Return Mocks --> PulumiEngine PulumiEngine -- 4. Return Mocks --> index.ts linkStyle 3 stroke-width:2px,fill:none,stroke:red; linkStyle 4 stroke-width:2px,fill:none,stroke:blue; linkStyle 5 stroke-width:2px,fill:none,stroke:blue
When Pulumi Mocks are not enough
There might be cases where Pulumi runtime mocks are not sufficient. E.g. we have a custom component with child resources and we want to assert them or we want to mock an external module over which we have no control. For that we can use the jest mocking capabilities https://jestjs.io/docs/mock-functions
Writing our first tests 🎉
Now that we understood how Pulumi Mocks work, we are ready to start writing our tests.
mkdir tests
touch tests/static-website.test.ts
Open static-website.test.ts
and add the following code to setup the mocks:
import * as pulumi from "@pulumi/pulumi";
pulumi.runtime.setMocks({
newResource: function(args: pulumi.runtime.MockResourceArgs): {id: string, state: any} {
return {
id: args.inputs.name + "_id",
state: args.inputs,
};
},
call: function(args: pulumi.runtime.MockCallArgs) {
return args.inputs;
},
});
Pulumi will invoke:
newResource
on each Resource Constructor and return a mocked resource with:id
in the formatargs.inputs.name + "_id"
state
that will contain all the inputs of that resource, so we will be able to assert on their values.
call
on each provider function call and return the inputs that were given.
Loading the Infrastructure under Test
We have to ensure loading our infrastructure code from index.ts before any tests but after the mocks are defined.Add the following code after the
pulumi.runtime.setMocks
block.
describe("When provisioning a static website", () => {
let infra: typeof import("../index");
beforeAll(async function() {
// It's important to import the program _after_ the mocks are defined.
infra = await import("../index");
})
});
Run npm build to validate everything is setup correctly:
npm run build
Test1: One of the requirements is to host the static website in a Storage Account, so let’s test that our code creates a storage account.
describe("When provisioning a static website", () => {
...
it("A storage account should be created", () => {
expect(infra.storageAccount).toBeDefined();
});
});
Run the tests:
npm run unit-tests
The test fails as expected, now we come into the TDD cycle, so let’s write enough code to pass the test. Add the following code to create a resource group and a storage account to index.ts:
import * as azure_native from "@pulumi/azure-native";
const rg = new azure_native.resources.ResourceGroup("static-website-rg");
export const storageAccount = new azure_native.storage.StorageAccount("staticwebsite", {
resourceGroupName: rg.name,
sku: {
name: azure_native.storage.SkuName.Standard_ZRS
},
kind: azure_native.storage.Kind.StorageV2,
});
Test2: Let’s add a test for making sure the storage account only allows https traffic
it("The storage account should only allow https traffic", function (done) {
infra.storageAccount.enableHttpsTrafficOnly.apply(enableHttpsTrafficOnly => {
if (!enableHttpsTrafficOnly) {
done(new Error(`storageAccount.enableHttpsTrafficOnly should be true`));
} else {
done();
}
});
});
enableHttpsTrafficOnly
is an output property, thus we need to use the apply method to get access to its value. Since all outputs are resolved asynchronously, we need to use the framework’s built-in asynchronous test capability.
Also this test will initially fail, to make it pass add enableHttpsTrafficOnly: true
to the storage account parameters in `index.ts``
Test3: Check that a StorageAccountStaticWebsite is created
it("A Storage Account Static Website should be created", () => {
expect(infra.staticWebsite).toBeDefined();
});
and the code to pass the test:
export const staticWebsite = new azure_native.storage.StorageAccountStaticWebsite("staticWebsite", {
accountName: storageAccount.name,
resourceGroupName: rg.name,
indexDocument: "index.html",
error404Document: "404.html",
});
Test4: Check that the StorageAccountStaticWebsite is using correct StorageAccount
— to be continued —